1. 데이터베이스 백업
- 백업을 위한 명령어 : mongodump
• dumps the entire data of your server into the dump directory
(서버의 전체 데이터를 덤프 디렉토리에 덤프)
• 백업 단위 선택 가능 : 컬렉션/데이터베이스/전체 데이터베이스 단위
ex) mongod 서버가 로컬호스트(포트# : 27017)에서 동작 중인 경우
• mongodb가 설치된 bin 폴더로 이동 후 mongodump 명령 입력
• 모든 데이터를 BSON 파일 형식으로 /bin/dump 폴더에 백업 수행
- mongodump와 함께 쓰는 옵션
Syntax | Description | Example |
mongodump --host HOST_NAME --port PORT_NUMBER |
This commmand will backup all databases of specified mongod instance. (지정된 mongod 인스턴스의 모든 데이터베이스를 백업) |
mongodump --host tutorialspoint.com --port 27017 |
mongodump --dbpath DB_PATH --out BACKUP_DIRECTORY |
This command will backup only specified database at specified path. ( 지정된 경로에서 지정된 데이터베이스 만 백업) |
mongodump --dbpath /data/db/ --out /data/backup/ |
mongodump --collection COLLECTION --db DB_NAME |
This command will backup only specified collection of specified database. (지정된 데이터베이스 모음만 백업) |
mongodump --collection mycol --db test |
2. 백업된 데이터 복원(restore) 명령 : mongorestore
• restores all of the data from the backup directory
##mongodump and mongostore are not ideal for capturing backups of larger systems.
(위 두 명령어는 큰 시스템의 백업 캡쳐에 적합하지 않다)
3. Relationship
• represents how various documents are logically related to each other
(다양한 문서가 서로 논리적으로 어떻게 연관되어 있는지 나타낸다)
• can be either 1:1, 1:N, N:1 or N:N
• can ne modeled via Embedded and Referenced approaches.
(embedded 및 referenced 접근 방식을 통해 모델링)
ex) 사용자의 주소 저장하는 경우 (여러 개의 주소 가능 -> 1:N 관계 성립)
{ "_id":ObjectId("52ffc33cd85242f436000001"),
"name": "Tom Hanks",
"contact": "987654321",
"dob": "01-01-1991" } //USER
{"_id":ObjectId("52ffc4a5d85242602e000000"),
"building": "22 A, Indiana Apt",
"pincode": 123456, "city": "Los Angeles",
"state": "California" } //Address
1) Embedded Relationships = denormalized relationship
• maintains all the related data in a single document, which makes it easy to retrieve and maintain
(모든 관련 데이터를 단일 문서로 유지 관리하여 쉽게 사용할 수 있도록 한다(회수 하여 유지))
{
"_id":ObjectId("u001"),
"name": "Tom Hanks", . . .
"address": [ { "building": "22 A, Indiana Apt", . . . },
{ "building": "170 A, Acropolis Apt", . . . } ] }
]
}
•whole document can be retrieved in a single query
(전체 문서는 단일 쿼리로 검색할 수 있다)
db.users.findOne({"name":"Tom Hanks"},{"address":1})
• drawback(단점)
– if the embedded document keeps on growing too much in size, it can impact the read/write performance.
(포함된 문서의 크기가 계속 커지면 읽기 / 쓰기 성능에 영향을 미칠 수 있다)
2) Referenced Relationships (참조 관계)= normalized relationship(정상화 관계)
• both the user and address documents will be maintained separately but the user document will contain a field that will reference the address document's id field.
(사용자 문서와 주소 문서는 별도로 유지되지만, 사용자 문서에는 주소 문서의 ID 필드를 참조하는 필드가 포함)
{
"_id":ObjectId("u001"),
. . .
"name": "Tom Hanks",
"address_ids": [ ObjectId("a001"), ObjectId("a002") ]
}
• need two queries: first to fetch the address_ids fields from user document and second to fetch these addresses from address collection.
(두 가지 쿼리가 필요 / 첫째는 사용자 문서에서 address_ids 필드를 가져오는 것 => 둘 째는 주소 집합에서 이 주소를 가져오는 것)
> var result = db.users.findOne({"name":"Tom Hanks"}, {"address_ids":1})
> db.address.find({"_id":{"$in":result["address_ids"] }})
//result 문서에서 address_ids 필드의 값만 추출 [ObjectId("a001"), ObjectId("a002")]
4. Database References
1) For many use cases in MongoDB(몽고디비의 많은 사용 사례)
• the denormalized data model where related data is stored within a single document will be optimal (embedded reltaionship)(관련 데이터가 저장되는 공식화된 데이터 모델 : 단일 문서가 최적 / 임베디드 Relationship)
• in some cases, it makes sense to store related information in separate documents, typically in different collections or databases (normalized relationship)
(경우에 따라 관련 정보를 별도로 저장하는 것이 타당 / 일반적으로 서로 다른 컬렉션 또는 DB 에 있는 문서(정규화된 관계))
2) two methods for relating documents in mongoDB applications(몽고디비 응용프로그램에서 Document와 관련된 2가지)
• Manual references(수동 참조)
– you save the _id field of one document in another document as a reference.
Then your application can run a second query to return the related data.
(하나의 document에 id 필드를 저장하고 다른 document에서 참조하면 된다
/ 응용프로그램에서 두 번째 쿼리를 실행하여 반환할 수 있다)
– simple and sufficient method for most use cases.
(대부분의 사용 사례에 대한 간단하고 충분한 방법)
• DBRefs
– references from one document to another using the value of the first document‟s _id field, collection name, and, optionally, its database name
(dbrefs를 사용하여 한 문서에서 다른 문서로 참조 / _id 필드, 컬렉션 이름 및 데이터베이스 이름(선택 사항) 등이 있다)
– in cases where a document contains references from different collections, we can use this method
(document에 다른 collection이 포함되어 있다면 , 위의 방법을 사용한다)
3) Using DBRefs
• $ref 필드 : 참조되는 문서가 속한 컬렉션 이름↓
• $id 필드 : 참조되는 문서의 _id 필드↓
• $db 필드 : 참조되는 문서가 속한 데이터베이스 이름 (옵션)↓
아래 방향으로 필드 순서 주의 해서 사용해야한다.
{
"_id":ObjectId("53402597d852426020000002"),
"address": {
"$ref": "address_home",
"$id": ObjectId("534009e4d852427820000002"),
"$db": "tutorialspoint"},
"contact": "987654321",
"dob": "01-01-1991",
"name": "Tom Hanks"
}
> var user = db.users.findOne({"name":"Tom Hanks"})
> var dbRef = user.address
> db[dbRef.$ref].findOne({"_id":(dbRef.$id)})
5. Covered Queries
1) covered query
• 인덱스만 이용해서도 처리가 가능한 질의 (데이터베이스 접근 없음)
• a query that can be satisfied entirely using an index and does not have to examine any documents
(인덱스를 사용하여 완전히 만족할 수 있고, 그 어떤 document도 검토할 필요가 없는 질의)
• 질의 처리에 필요한 모든 데이터가 인덱스에 있을 때 „이 인덱스가 해당 질의를 커버 한다‟라고 함
• An index covers a query when all of the following apply:(모두 적용되면 index는 query를 다룬다)
– all the fields in the query are part of an index, and(쿼리의 모든 필드는 index의 일부분)
– all the fields returned in the results are in the same index(결과에 반환되는 모든 필드가 동일한 index에 있음)
– no fields in the query are equal to null(쿼리에 null과 동일한 필드가 없음)
{
"_id": ObjectId("53402597d852426020000002"),
"contact": "987654321",
"dob": "01-01-1991",
"gender": "M",
"name": "Tom Benzamin",
"user_name": "tombenzamin"
}
First. create a compound index for the users collection on the fields gender and user_name using the following query
>db.users.ensureIndex({gender:1,user_name:1})
this index will cover the following query
>db.users.find({gender:"M"},{user_name:1,_id:0}) //ID필드는 결과 문서에서 반드시 제외시켜야 함
6. Analyzing Queries
- Analyzing queries is a very important aspect of measuring how effective the database and indexing design is.
(쿼리 분석하는 것은 효과적으로 데이터베이스와 인덱스를 디자인하는데 있어 매우 중요한 측면)
1) $explain( ) 연산자
• provides information on the query, indexes used in a query and other statistics
(쿼리에 대한 정보, 쿼리에 사용된 인덱스 및 기타 통계 제공)
• very useful when analyzing how well your indexes are optimized
(인덱스가 얼마나 최적화 되어있는지 분석할 때 유용)
• 사용 예)
> db.users.find({gender:"M"},{name:1, _id:0}).explain()
결과
>{ "cursor" : "BtreeCursor gender_1_user_name_1", "isMultiKey" : false, "n" : 1, "nscannedObjects" : 0, "nscanned" : 1, "nscannedObjectsAllPlans" : 0, "nscannedAllPlans" : 1, "scanAndOrder" : false, "indexOnly" : true, "nYields" : 0, "nChunkSkips" : 0, "millis" : 0, "indexBounds" : { "gender" : [ [ "M", "M" ] ], "user_name" : [ [ { "$minElement" : 1 }, { "$maxElement" : 1 } ] ] } }
2) $hint( ) 연산자
• forces the query optimizer to use the specified index to run a query
(쿼리 최적화가 지정된 인덱스를 사용하여 쿼리를 실행하도록 강제 적용)
• particularly useful when you want to test performance of a query with different indexes
(다른 인덱스로 쿼리 성능을 테스트하는데 유용)
• 사용 예)
>db.users.find({gender:"M"},{user_name:1,_id:0}).hint({gender:1,user_name:1})
7. Atomic Operations
1) MongoDB provides atomic operations on only a single document
(몽고디비는 단일 document)에서만 atomic operations 을 제공
2) The recommended approach to maintain atomicity(원자성 유지하기 위한 권장하는 접근법)
• keep all the related information, which is frequently updated together in a single document using embedded documents(임베디드 문서를 사용하여 단일 문서로 자주 업데이트 되는 모든 관련 정보를 보관)
=> all the updates for a single document are atomic.(단일 document 에 대한 모든 업데이트는 원자적)
• findAndModify 명령의 활용 예)
{ "_id":1,
"product_name": "Samsung S3",
"product_available": 3,
"product_bought_by": [{ "customer": "john", "date": "7-Jan-2014"}, { "customer": "mark", "date": "8-Jan-2014"} ] }
> db.products.findAndModify({
query:{_id:2,product_available:{$gt:0}},
update:{ $inc:{product_available:-1},
$push:{product_bought_by:{customer:"rob",date:"9-Jan-2014"}} }
})
8. Advanced Indexing
{
"address": {
"city": "Los Angeles",
"state": "California",
"pincode": "123"
},
"tags": [
"music",
"cricket",
"blogs"
],
"name": "Tom Benzamin"
}
1) 배열을 필드 값으로 가지는 인덱싱 : multikey index 로 구성
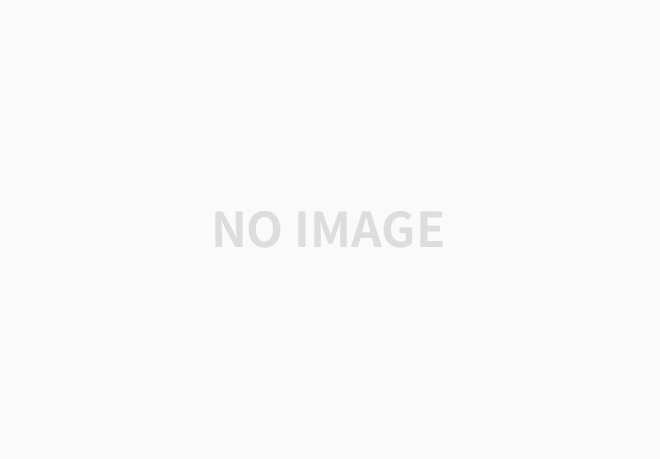
– 배열의 각 요소 마다 인덱스 엔트리 유지
=> 같은 배열에서 생성된 여러 엔트리들은 동일한 문서를 참조(포인트)
– user tag를 기반으로 user document를 검색한다 가정 / collection의 tag 배열에 index를 생성
– 배열에 index 작성 시 , 각 필드에 대한 별도 index 항목이 작성
==> 태그 배열에서 index 생성 시, music/ cricket / blogs 값에 대해 별도의 index 생성
> db.users.createIndex({"tags":1})
//태그 배열에 index 생성
> db.users.find({"tags":"cricket"})
//index 생성 후 컬렉션의 태그 필드 검색
> db.users.find({"tags":"cricket"}).explain()
//적절하게 사용되었는지 확인하려면 explain 명령 사용
"cursor": "BtreeCursor tags_1"//결과
2) 서브 문서를 필드 값으로 갖는 인덱싱
– 서브 문서의 모든 필드들에 대한 인덱스 생성 => compound index로 구성
– 도시 , 주 및 핀코드 필드 기반 문서 검색 /
=> 모든 필드는 주소, 하위 문서 필드의 일부 이므로 -> 하위 문서의 모든 필드에 대한 index 생성
db.users.createIndex({"addr.city":1},{"addr.state":1}, {"addr.pincode":1})
//하위 문서의 세 필드 모두에 대한 index
db.users.find({"addr.city":"LA"})
//index을 사용하는 하위 문서 필드 검색 가능
db.users.find({"addr.city":"LA", "addr.state":1})
//쿼리 식은 지정된 index 순서를 따라야함
db.users.find({"addr.city":"LA", "addr.state":1, "addr.pincode":1})
'Database Study > MongoDB' 카테고리의 다른 글
[Mongodb]웹서비스컴퓨팅_11주차_(1)_Sharding (0) | 2019.12.07 |
---|---|
[Mongodb]웹서비스컴퓨팅_10주차 (0) | 2019.12.03 |
[Mongodb]웹서비스컴퓨팅_9주차 (0) | 2019.12.03 |
[Mongodb]웹서비스컴퓨팅_7주차 (0) | 2019.10.15 |
[Mongodb]웹서비스컴퓨팅_6주차 (0) | 2019.10.01 |